I know many of you are just looking for some reference code to copy so I put that here at the top, but if you want to learn more, a detailed explanation can be found in the video above and in the text below.
import sys
from PyQt5.QtWidgets import (QApplication, QMainWindow, QVBoxLayout,
QWidget, QLineEdit, QLabel, QPushButton)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("My QLineEdit Window")
main_layout = QVBoxLayout()
# 1. Creating
self.line_edit1 = QLineEdit()
main_layout.addWidget(self.line_edit1)
# 2. Placeholder Text
self.line_edit1.setPlaceholderText("Enter text here...")
# 3. Getting the text
self.get_button = QPushButton("Get text in line edit 1")
main_layout.addWidget(self.get_button)
self.get_button.clicked.connect(self.get_button_pressed)
self.gotten_text_label = QLabel("Text gotten: ")
main_layout.addWidget(self.gotten_text_label)
# 4. Setting text
self.set_button = QPushButton('Press to set line edit 1 to "cat"')
main_layout.addWidget(self.set_button)
self.set_button.clicked.connect(self.set_button_pressed)
# 5. textEdited Callback
self.text_edited_label = QLabel("textEdit callback: ")
main_layout.addWidget(self.text_edited_label)
self.line_edit1.textEdited.connect(self.line1_text_edited)
# 6. textChanged Callback
self.text_changed_label = QLabel("textChanged Callback: ")
main_layout.addWidget(self.text_changed_label)
self.line_edit1.textChanged.connect(self.line1_text_changed)
# 7. Signal when editing is finished
self.line_edit2 = QLineEdit()
self.editing_finished_label = QLabel()
self.return_pressed_label = QLabel()
main_layout.addWidget(self.line_edit2)
main_layout.addWidget(self.editing_finished_label)
main_layout.addWidget(self.return_pressed_label)
self.line_edit1.editingFinished.connect(self.line1_editing_finished)
self.line_edit1.returnPressed.connect(self.line1_return_pressed)
widget = QWidget()
widget.setLayout(main_layout)
self.setCentralWidget(widget)
def get_button_pressed(self):
text = self.line_edit1.text()
self.gotten_text_label.setText("Text gotten: " + text)
def set_button_pressed(self):
self.line_edit1.setText("cat")
def line1_text_edited(self, text):
self.text_edited_label.setText("textEdit callback: " + text)
def line1_text_changed(self, text):
self.text_changed_label.setText("textChanged Callback: " + text)
def line1_return_pressed(self):
self.return_pressed_label.setText("Return Pressed")
def line1_editing_finished(self):
self.editing_finished_label.setText("Editing Finished")
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
app.exec()
if __name__ == '__main__':
main()
Boilerplate Code
For us to work with QLineEdit
s, we will need to setup a window to quickly do that. The code for which can be found below:
import sys
from PyQt5.QtWidgets import (QApplication, QMainWindow, QVBoxLayout,
QWidget)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("My QLineEdit Window")
main_layout = QVBoxLayout()
widget = QWidget()
widget.setLayout(main_layout)
self.setCentralWidget(widget)
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
app.exec()
if __name__ == '__main__':
main()
Starting off at the top, we have some imports for the things we will need.
Lines 5-8, subclass QMainWindow
in order to allow us to create our own custom main window to add content to. Then it initializes the class by running the super class’ init()
method, and setting the title for the window to "My QLineEdit Window"
.
Line 9 created a QVBoxLayout. Lines 11-13 create a widget, adds the layout to that widget and sets it as the central widget for this application.
If you haven’t worked with layouts before, I have a tutorial covering them, but for now all you really need to know is that this line, and the lines below it make it so you can add widgets (like labels and push buttons) to your interface so that they are stacked vertically and stretch nicely when the window is resized.
Lines 16-20 define the main()
function which creates an application initialized with the given command line arguments (sys.argv
), creates a main window from our custom class, shows the window, and executes the application.
Lastly, lines 23 and 24 runs the main()
function if this is the main file being run.
If you save the file and run it, it should look like this:
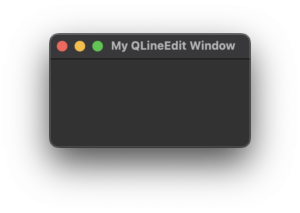
Now let’s get some user input!
Creating a LineEdit
Starting off, we need to update line 3 to import QLineEdit
:
from PyQt5.QtWidgets import (QApplication, QMainWindow, QVBoxLayout,
QWidget, QLineEdit)
On line 12 we are going to create a QLineEdit
in the variable self.line_edit1
. Then, on line 13 we add it to the layout.
# 1. Creating
self.line_edit1 = QLineEdit()
main_layout.addWidget(self.line_edit1)
Click here for the full code example…
import sys
from PyQt5.QtWidgets import (QApplication, QMainWindow, QVBoxLayout,
QWidget, QLineEdit)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("My QLineEdit Window")
main_layout = QVBoxLayout()
# 1. Creating
self.line_edit1 = QLineEdit()
main_layout.addWidget(self.line_edit1)
widget = QWidget()
widget.setLayout(main_layout)
self.setCentralWidget(widget)
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
app.exec()
if __name__ == '__main__':
main()
And if we run that code we should get:
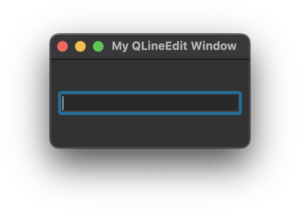
Placeholder Text
It is useful to be able to prompt the user as to what is supposed to be entered in the QLineEdit so lets add some placeholder text!
This is done by running setPlaceholderText()
on the line edit passing in the text you want it to be:
main_layout.addWidget(self.line_edit1)
# 2. Placeholder Text
self.line_edit1.setPlaceholderText("Enter text here...")
Click here for the full code example…
import sys
from PyQt5.QtWidgets import (QApplication, QMainWindow, QVBoxLayout,
QWidget, QLineEdit)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("My QLineEdit Window")
main_layout = QVBoxLayout()
# 1. Creating
self.line_edit1 = QLineEdit()
main_layout.addWidget(self.line_edit1)
# 2. Placeholder Text
self.line_edit1.setPlaceholderText("Enter text here...")
widget = QWidget()
widget.setLayout(main_layout)
self.setCentralWidget(widget)
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
app.exec()
if __name__ == '__main__':
main()
And if we run that code we should get:

Getting the Text
Next we are going to look at getting the text.
To exemplify this, we are going to create a QPushButton
, and a QLabel
. When the user clicks on the QPushButton
, it will update the QLabel
to have the text from line_edit1
.
If you haven’t worked with QPushButton
s before, I have an article that covers them here. Similarly, for QLabel
s, I have an article here.
Starting off at the top, we need to update our imports to include QPushButton and QLabel:
import sys
from PyQt5.QtWidgets import (QApplication, QMainWindow, QVBoxLayout,
QWidget, QLineEdit, QPushButton, QLabel)
Then we need to create our pushbutton, and label, add them to the layout, and register a callback button which we will call self.get_button_pressed
:
self.line_edit1.setPlaceholderText("Enter text here...")
# 3. Getting the text
self.get_button = QPushButton("Get text in line edit 1")
main_layout.addWidget(self.get_button)
self.get_button.clicked.connect(self.get_button_pressed)
self.gotten_text_label = QLabel("Text gotten: ")
main_layout.addWidget(self.gotten_text_label)
Then, under the init()
we define the function:
self.setCentralWidget(widget)
def get_button_pressed(self):
text = self.line_edit1.text()
self.gotten_text_label.setText("Text gotten: " + text)
We get self.line_edit1
‘s text by running the text()
method on it. We then update self.gotten_text_label
with the setText()
method.
Click here for the full code example…
import sys
from PyQt5.QtWidgets import (QApplication, QMainWindow, QVBoxLayout,
QWidget, QLineEdit, QPushButton, QLabel)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("My QLineEdit Window")
main_layout = QVBoxLayout()
# 1. Creating
self.line_edit1 = QLineEdit()
main_layout.addWidget(self.line_edit1)
# 2. Placeholder Text
self.line_edit1.setPlaceholderText("Enter text here...")
# 3. Getting the text
self.get_button = QPushButton("Get text in line edit 1")
main_layout.addWidget(self.get_button)
self.get_button.clicked.connect(self.get_button_pressed)
self.gotten_text_label = QLabel("Text gotten: ")
main_layout.addWidget(self.gotten_text_label)
widget = QWidget()
widget.setLayout(main_layout)
self.setCentralWidget(widget)
def get_button_pressed(self):
text = self.line_edit1.text()
self.gotten_text_label.setText("Text gotten: " + text)
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
app.exec()
if __name__ == '__main__':
main()
And if we run that code we should get:
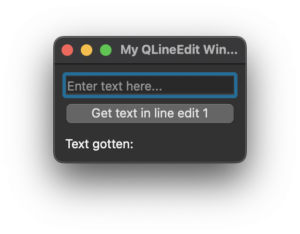
And if we type “hello” in the line edit, and push the bottom button, the label should be updated:
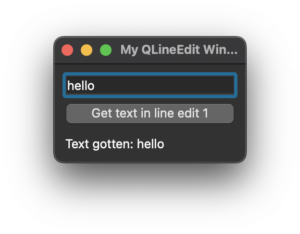
Setting Text in a QLineEdit
Sometimes it can be useful to update the text in a QLineEdit programmatically. Lets get into how to do this.
We are going to create a new button that will set the text in the line edit to “cat”.
main_layout.addWidget(self.gotten_text_label)
# 4. Setting text
self.set_button = QPushButton('Press to set line edit 1 to "cat"')
main_layout.addWidget(self.set_button)
self.set_button.clicked.connect(self.set_button_pressed)
On line 28, we create a new button called self.set_button. We then add it to the layout and create a new callback to the function self.set_button_pressed
, for when the button is clicked.
Then later we define the function.
self.gotten_text_label.setText("Text gotten: " + text)
def set_button_pressed(self):
self.line_edit1.setText("cat")
All we do in the function is run setText()
in self.line_edit1
passing it the text we want to set, “cat"
.
Click here for the full code example…
import sys
from PyQt5.QtWidgets import (QApplication, QMainWindow, QVBoxLayout,
QWidget, QLineEdit, QPushButton, QLabel)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("My QLineEdit Window")
main_layout = QVBoxLayout()
# 1. Creating
self.line_edit1 = QLineEdit()
main_layout.addWidget(self.line_edit1)
# 2. Placeholder Text
self.line_edit1.setPlaceholderText("Enter text here...")
# 3. Getting the text
self.get_button = QPushButton("Get text in line edit 1")
main_layout.addWidget(self.get_button)
self.get_button.clicked.connect(self.get_button_pressed)
self.gotten_text_label = QLabel("Text gotten: ")
main_layout.addWidget(self.gotten_text_label)
# 4. Setting text
self.set_button = QPushButton('Press to set line edit 1 to "cat"')
main_layout.addWidget(self.set_button)
self.set_button.clicked.connect(self.set_button_pressed)
widget = QWidget()
widget.setLayout(main_layout)
self.setCentralWidget(widget)
def get_button_pressed(self):
text = self.line_edit1.text()
self.gotten_text_label.setText("Text gotten: " + text)
def set_button_pressed(self):
self.line_edit1.setText("cat")
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
app.exec()
if __name__ == '__main__':
main()
And if we run that code we should get:
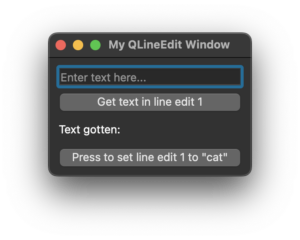
And if we push the bottom button, "cat"
is added to the line edit:
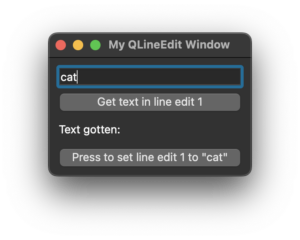
textEdited Callback
Sometimes we want to get the text as it is being typed. We can do this by attaching a callback to the textEdited
signal. To show this, we are going to create a new label and update it every time the user updates the line edit.
self.set_button.clicked.connect(self.set_button_pressed)
# 5. textEdited Callback
self.text_edited_label = QLabel("textEdit callback: ")
main_layout.addWidget(self.text_edited_label)
self.line_edit1.textEdited.connect(self.line1_text_edited)
Starting on line 32, we create a QLabel
, add it to the layout and set the callback for self.line_edit1
‘s textEdited
signal to be the function self.line1_text_edited
.
Then, below the init() method, we define the self.line1_text_edited
() method:
self.line_edit1.setText("cat")
def line1_text_edited(self, text):
self.text_edited_label.setText("textEdit callback: " + text)
Unlike some of the other callback functions we have used so far, this one has a parameter which we named text
, which is the updated text from self.line_edit1
. We then set our newly created label to show that text.
Click here for the full code example…
import sys
from PyQt5.QtWidgets import (QApplication, QMainWindow, QVBoxLayout,
QWidget, QLineEdit, QPushButton, QLabel)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("My QLineEdit Window")
main_layout = QVBoxLayout()
# 1. Creating
self.line_edit1 = QLineEdit()
main_layout.addWidget(self.line_edit1)
# 2. Placeholder Text
self.line_edit1.setPlaceholderText("Enter text here...")
# 3. Getting the text
self.get_button = QPushButton("Get text in line edit 1")
main_layout.addWidget(self.get_button)
self.get_button.clicked.connect(self.get_button_pressed)
self.gotten_text_label = QLabel("Text gotten: ")
main_layout.addWidget(self.gotten_text_label)
# 4. Setting text
self.set_button = QPushButton('Press to set line edit 1 to "cat"')
main_layout.addWidget(self.set_button)
self.set_button.clicked.connect(self.set_button_pressed)
# 5. textEdited Callback
self.text_edited_label = QLabel("textEdit callback: ")
main_layout.addWidget(self.text_edited_label)
self.line_edit1.textEdited.connect(self.line1_text_edited)
widget = QWidget()
widget.setLayout(main_layout)
self.setCentralWidget(widget)
def get_button_pressed(self):
text = self.line_edit1.text()
self.gotten_text_label.setText("Text gotten: " + text)
def set_button_pressed(self):
self.line_edit1.setText("cat")
def line1_text_edited(self, text):
self.text_edited_label.setText("textEdit callback: " + text)
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
app.exec()
if __name__ == '__main__':
main()
And if we run that code we should get:
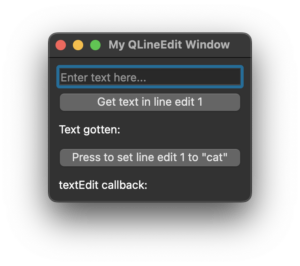
And if we type "hello"
in the line edit, it should look like this:
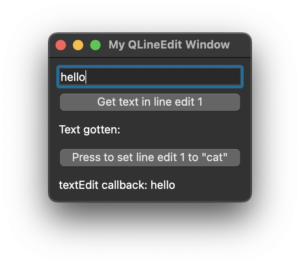
textChanged Callback
Going back to our textEdited
example we just finished, you will notice that if we press the bottom button, it will still update the line edit to have the text "cat"
, but the bottom label will not be updated. That is because the textEdited
signal is not sent, and its corresponding callback is not called when the line edit is updated programmatically instead of directly by the user.
Sometimes it can be useful to be able to call a function whenever the line edit is updated, whether that is directly by the user or programmatically. We can do that with the textChanged signal. Like our previous example, we will create a new label and update that when the textChanged callback function is called.
self.line_edit1.textEdited.connect(self.line1_text_edited)
# 6. textChanged Callback
self.text_changed_label = QLabel("textChanged Callback: ")
main_layout.addWidget(self.text_changed_label)
self.line_edit1.textChanged.connect(self.line1_text_changed)
Starting on line 39, we create a QLabel
, add it to the layout and set the callback for self.line_edit1
‘s textChanged
signal to be the function self.line1_text_edited
.
Then, below the init() method, we define the self.line1_text_changed
() method:
self.text_edited_label.setText("textEdit callback: " + text)
def line1_text_changed(self, text):
self.text_changed_label.setText("textChanged Callback: " + text)
Like with textEdited
, this function has a parameter which we named text
, which is the updated text from self.line_edit1
. We then set our newly created label to show that text.
Click here for the full code example…
import sys
from PyQt5.QtWidgets import (QApplication, QMainWindow, QVBoxLayout,
QWidget, QLineEdit, QPushButton, QLabel)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("My QLineEdit Window")
main_layout = QVBoxLayout()
# 1. Creating
self.line_edit1 = QLineEdit()
main_layout.addWidget(self.line_edit1)
# 2. Placeholder Text
self.line_edit1.setPlaceholderText("Enter text here...")
# 3. Getting the text
self.get_button = QPushButton("Get text in line edit 1")
main_layout.addWidget(self.get_button)
self.get_button.clicked.connect(self.get_button_pressed)
self.gotten_text_label = QLabel("Text gotten: ")
main_layout.addWidget(self.gotten_text_label)
# 4. Setting text
self.set_button = QPushButton('Press to set line edit 1 to "cat"')
main_layout.addWidget(self.set_button)
self.set_button.clicked.connect(self.set_button_pressed)
# 5. textEdited Callback
self.text_edited_label = QLabel("textEdit callback: ")
main_layout.addWidget(self.text_edited_label)
self.line_edit1.textEdited.connect(self.line1_text_edited)
# 6. textChanged Callback
self.text_changed_label = QLabel("textChanged Callback: ")
main_layout.addWidget(self.text_changed_label)
self.line_edit1.textChanged.connect(self.line1_text_changed)
widget = QWidget()
widget.setLayout(main_layout)
self.setCentralWidget(widget)
def get_button_pressed(self):
text = self.line_edit1.text()
self.gotten_text_label.setText("Text gotten: " + text)
def set_button_pressed(self):
self.line_edit1.setText("cat")
def line1_text_edited(self, text):
self.text_edited_label.setText("textEdit callback: " + text)
def line1_text_changed(self, text):
self.text_changed_label.setText("textChanged Callback: " + text)
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
app.exec()
if __name__ == '__main__':
main()
And if we run that code we should get:

And if we type "hello"
in the line edit, it should look like this:

But if we press the bottom button, we will see the textChanged
label be updated but not the textEdited
:
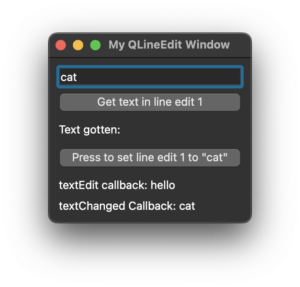
Signals for When Editing is Done
Lastly, I am going to introduce you to two more signals: editingFinished and returnPressed. It can be useful to know when a user is done editing a line edit. The signal returnPressed is emitted, as you can probably guess, when the user is editing a line edit, and hits the return or enter button. The signal editingFinished is emitted when either enter is pressed or the line edit loses focus. Losing focus happens when the user clicks on another widget that can take focus.
We are going to show these signals at work by creating a new QLineEdit so we can change focus, and two new labels which will be updated when their corresponding signal is emitted.
self.line_edit1.textChanged.connect(self.line1_text_changed)
# 7. Signal when editing is finished
self.line_edit2 = QLineEdit()
self.editing_finished_label = QLabel()
self.return_pressed_label = QLabel()
main_layout.addWidget(self.line_edit2)
main_layout.addWidget(self.editing_finished_label)
main_layout.addWidget(self.return_pressed_label)
self.line_edit1.editingFinished.connect(self.line1_editing_finished)
self.line_edit1.returnPressed.connect(self.line1_return_pressed)
Starting on line 42, we create a QLineEdit and two QLabel
s, we add them to the layout, and set the callback functions for self.line_edit1
‘s editingFinished
, and returnPressed
signals.
Then, below the init() method, we define the functions:
self.text_changed_label.setText("textChanged Callback: " + text)
def line1_return_pressed(self):
self.return_pressed_label.setText("Return Pressed")
def line1_editing_finished(self):
self.editing_finished_label.setText("Editing Finished")
In these functions, we update the corresponding labels to tell us when each of the signals are emitted.
Click here for the full code example…
import sys
from PyQt5.QtWidgets import (QApplication, QMainWindow, QVBoxLayout,
QWidget, QLineEdit, QLabel, QPushButton)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("My QLineEdit Window")
main_layout = QVBoxLayout()
# 1. Creating
self.line_edit1 = QLineEdit()
main_layout.addWidget(self.line_edit1)
# 2. Placeholder Text
self.line_edit1.setPlaceholderText("Enter text here...")
# 3. Getting the text
self.get_button = QPushButton("Get text in line edit 1")
main_layout.addWidget(self.get_button)
self.get_button.clicked.connect(self.get_button_pressed)
self.gotten_text_label = QLabel("Text gotten: ")
main_layout.addWidget(self.gotten_text_label)
# 4. Setting text
self.set_button = QPushButton('Press to set line edit 1 to "cat"')
main_layout.addWidget(self.set_button)
self.set_button.clicked.connect(self.set_button_pressed)
# 5. textEdited Callback
self.text_edited_label = QLabel("textEdit callback: ")
main_layout.addWidget(self.text_edited_label)
self.line_edit1.textEdited.connect(self.line1_text_edited)
# 6. textChanged Callback
self.text_changed_label = QLabel("textChanged Callback: ")
main_layout.addWidget(self.text_changed_label)
self.line_edit1.textChanged.connect(self.line1_text_changed)
# 7. Signal when editing is finished
self.line_edit2 = QLineEdit()
self.editing_finished_label = QLabel()
self.return_pressed_label = QLabel()
main_layout.addWidget(self.line_edit2)
main_layout.addWidget(self.editing_finished_label)
main_layout.addWidget(self.return_pressed_label)
self.line_edit1.editingFinished.connect(self.line1_editing_finished)
self.line_edit1.returnPressed.connect(self.line1_return_pressed)
widget = QWidget()
widget.setLayout(main_layout)
self.setCentralWidget(widget)
def get_button_pressed(self):
text = self.line_edit1.text()
self.gotten_text_label.setText("Text gotten: " + text)
def set_button_pressed(self):
self.line_edit1.setText("cat")
def line1_text_edited(self, text):
self.text_edited_label.setText("textEdit callback: " + text)
def line1_text_changed(self, text):
self.text_changed_label.setText("textChanged Callback: " + text)
def line1_return_pressed(self):
self.return_pressed_label.setText("Return Pressed")
def line1_editing_finished(self):
self.editing_finished_label.setText("Editing Finished")
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
app.exec()
if __name__ == '__main__':
main()
And if we run that code we should get:
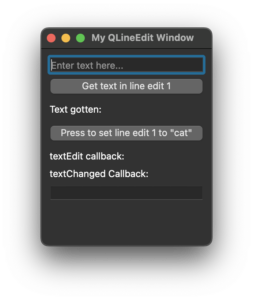
And if we type "hello"
in the top line edit, and then press return or enter, it should look like this:
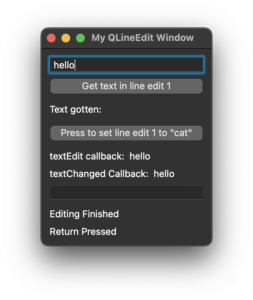
But if we close the program, rerun it, type in “hello” in the top line edit, and then click on the bottom line edit, we get:
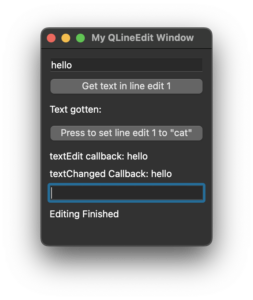
That is because the returnPressed
signal was not sent when you switched the focus by clicking on the lower line edit.
And those are the essentials of QLineEdit
s!