I know many of you are just looking for some reference code to copy so I put that here at the top, but if you want to learn more, a detailed explanation can be found in the video above and in the text below.
import sys
from PyQt5.QtWidgets import (QApplication, QMainWindow, QVBoxLayout, QWidget,
QCheckBox)
from PyQt5.QtCore import Qt
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("My QCheckBox Window")
main_layout = QVBoxLayout()
self.checkbox = QCheckBox("I like coding in Python")
main_layout.addWidget(self.checkbox)
self.checkbox.setChecked(True)
print("Checkbox state: {}".format(self.checkbox.isChecked()))
self.checkbox.stateChanged.connect(self.checkbox_updated)
widget = QWidget()
widget.setLayout(main_layout)
self.setCentralWidget(widget)
def checkbox_updated(self, state):
if state == Qt.CheckState.Checked:
print("Checkbox is checked")
else:
print("Checkbox is not checked")
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
app.exec()
if __name__ == '__main__':
main()
Boilerplate Code
For us to work with QCheckBox
es, we will need to setup a window to quickly do that. The code for which can be found below:
import sys
from PyQt5.QtWidgets import (QApplication, QMainWindow, QVBoxLayout,
QWidget)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("My QLineEdit Window")
main_layout = QVBoxLayout()
widget = QWidget()
widget.setLayout(main_layout)
self.setCentralWidget(widget)
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
app.exec()
if __name__ == '__main__':
main()
Starting off at the top, we have some imports for the things we will need.
Lines 5-8, subclass QMainWindow
in order to allow us to create our own custom main window to add content to. Then it initializes the class by running the super class’ init()
method, and setting the title for the window to "My QLineEdit Window"
.
Line 9 created a QVBoxLayout. Lines 11-13 create a widget, adds the layout to that widget and sets it as the central widget for this application.
If you haven’t worked with layouts before, I have a tutorial covering them, but for now all you really need to know is that this line, and the lines below it make it so you can add widgets (like labels and push buttons) to your interface so that they are stacked vertically and stretch nicely when the window is resized.
Lines 16-20 define the main()
function which creates an application initialized with the given command line arguments (sys.argv
), creates a main window from our custom class, shows the window, and executes the application.
Lastly, lines 23 and 24 runs the main()
function if this is the main file being run.
If you save the file and run it, it should look like this:
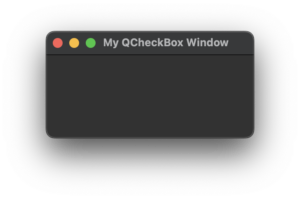
Now let’s check some boxes!
Creating a QCheckBox
Starting off, we need to update line 3 to import QCheckBox
:
from PyQt5.QtWidgets import (QApplication, QMainWindow, QVBoxLayout, QWidget,
QCheckBox)
On line 11 we are going to create a QCheckBox
in the variable self.checkbox
passing it the text we want next to the checkbox. Then, on line 12 we add it to the layout.
self.checkbox = QCheckBox("I like coding in Python")
main_layout.addWidget(self.checkbox)
Click here for the full code example…
import sys
from PyQt5.QtWidgets import (QApplication, QMainWindow, QVBoxLayout, QWidget,
QCheckBox)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("My QCheckBox Window")
main_layout = QVBoxLayout()
self.checkbox = QCheckBox("I like coding in Python")
main_layout.addWidget(self.checkbox)
widget = QWidget()
widget.setLayout(main_layout)
self.setCentralWidget(widget)
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
app.exec()
if __name__ == '__main__':
main()
And if we run that code we should get:
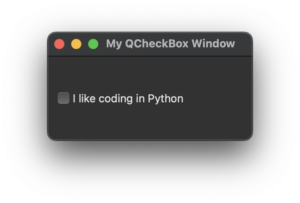
Setting the State
It can be useful to set the state of a checkbox as checked or unchecked.
It is pretty straightforward to do this. All we need to do is run the setChecked()
method on the checkbox, passing a boolean on whether want it to be checked or not. In our example we are passing it True
. On line 14, we add that to the code:
main_layout.addWidget(self.checkbox)
self.checkbox.setChecked(True)
Click here for the full code example…
import sys
from PyQt5.QtWidgets import (QApplication, QMainWindow, QVBoxLayout, QWidget,
QCheckBox)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("My QCheckBox Window")
main_layout = QVBoxLayout()
self.checkbox = QCheckBox("I like coding in Python")
main_layout.addWidget(self.checkbox)
self.checkbox.setChecked(True)
widget = QWidget()
widget.setLayout(main_layout)
self.setCentralWidget(widget)
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
app.exec()
if __name__ == '__main__':
main()
And if we run that code we should get:
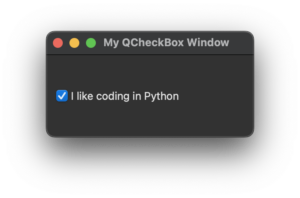
Getting the State
It can be useful to get the state of a checkbox.
This is also pretty straightforward. All we need to do is run the getChecked()
method on the checkbox. This will return a boolean telling us whether the checkbox is checked or not. In our example we are going to be printing out whether it is checked or not. On line 16, we add that to the code:
self.checkbox.setChecked(True)
print("Checkbox state: {}".format(self.checkbox.isChecked()))
Click here for the full code example…
import sys
from PyQt5.QtWidgets import (QApplication, QMainWindow, QVBoxLayout, QWidget,
QCheckBox)
from PyQt5.QtCore import Qt
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("My QCheckBox Window")
main_layout = QVBoxLayout()
self.checkbox = QCheckBox("I like coding in Python")
main_layout.addWidget(self.checkbox)
self.checkbox.setChecked(True)
print("Checkbox state: {}".format(self.checkbox.isChecked()))
widget = QWidget()
widget.setLayout(main_layout)
self.setCentralWidget(widget)
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
app.exec()
if __name__ == '__main__':
main()
And if we run that code, in the terminal we should see the following print out:
Checkbox state: True
Callback Function
Lastly, it can also be useful to run some code whenever a user clicks on a checkbox.
Starting on line 4, we add an import Qt
from QtCore
. This will be needed later.
import sys
from PyQt5.QtWidgets import (QApplication, QMainWindow, QVBoxLayout, QWidget,
QCheckBox)
from PyQt5.QtCore import Qt
Then on line 19, we connect self.checkbox
‘s stateChanged
signal to a new function we call self.checkbox_updated
:
print("Checkbox state: {}".format(self.checkbox.isChecked()))
self.checkbox.stateChanged.connect(self.checkbox_updated)
Below the init()
method, we define our function. In our example, we are going to print out if the checkbox is checked or not. The callback function is provided a parameter which we name state
. It gives the state of the checkbox as an integer.
In Qt, there can be three states for a checkbox. I’m not going to be covering why checkboxes can be tri-state but, we need the Qt
that we imported in order to see what that state is :
self.setCentralWidget(widget)
def checkbox_updated(self, state):
if state == Qt.CheckState.Checked:
print("Checkbox is checked")
else:
print("Checkbox is not checked")
Click here for the full code example…
import sys
from PyQt5.QtWidgets import (QApplication, QMainWindow, QVBoxLayout, QWidget,
QCheckBox)
from PyQt5.QtCore import Qt
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("My QCheckBox Window")
main_layout = QVBoxLayout()
self.checkbox = QCheckBox("I like coding in Python")
main_layout.addWidget(self.checkbox)
self.checkbox.setChecked(True)
print("Checkbox state: {}".format(self.checkbox.isChecked()))
self.checkbox.stateChanged.connect(self.checkbox_updated)
widget = QWidget()
widget.setLayout(main_layout)
self.setCentralWidget(widget)
def checkbox_updated(self, state):
if state == Qt.CheckState.Checked:
print("Checkbox is checked")
else:
print("Checkbox is not checked")
def main():
app = QApplication(sys.argv)
window = MainWindow()
window.show()
app.exec()
if __name__ == '__main__':
main()
If we run that code, and uncheck the checkbox , in the terminal, it should print out:
Checkbox is not checked
And if we check the checkbox , in the terminal, it should print out:
Checkbox is checked
And those are the essentials of QCheckBox
es!